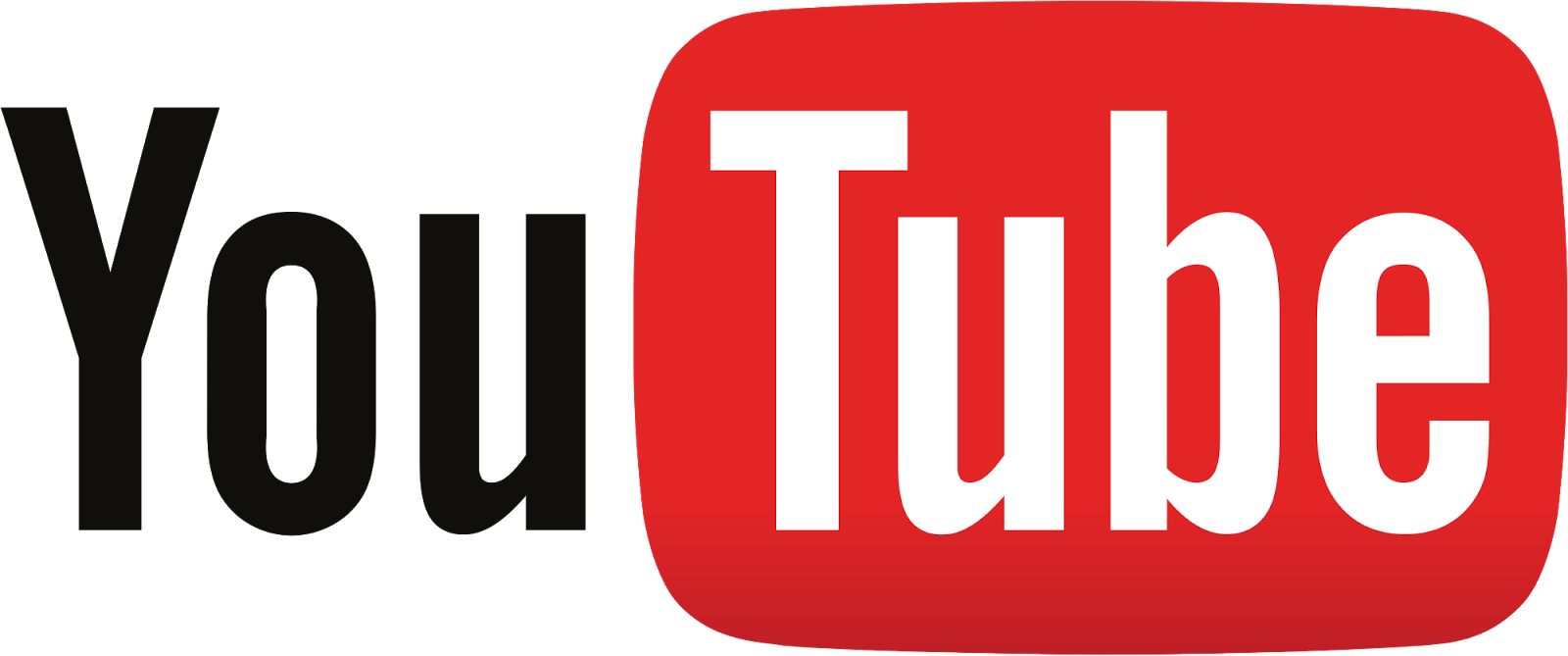
If you want to build a python script to get the result from the youtube based on the search query here is the script which you can run from a terminal and use in ubuntu.
For this, you will need the developer key for which you have to create an account but if you have a google account that's great then we are just a few clicks away from our developer key.
Before going into that just install this package click here
Just go to google developer projects page by clicking here and add a project for which you want to get the keys.
Then click on the project and go to Credentials on the left tab panel and then click create new key button.
It will ask you for which type of key, it will describe also that which type of key you are going to use on which kind.
So basically select the Browser key and you will be asked for the domains specification if you needed to restrict.
Lastly, press create.
You will have the keys on the project page which various other details will be along with it. So API KEY is the main key that we are going to use in our script.
After getting the key go through the process to install some pre-requirements from here and then here is the script which you can run from the terminal as python filename.py
#!/usr/bin/python from apiclient.discovery import build from apiclient.errors import HttpError from oauth2client.tools import argparser # Set DEVELOPER_KEY to the API key value from the APIs & auth > Registered apps # tab of # https://cloud.google.com/console # Please ensure that you have enabled the YouTube Data API for your project. DEVELOPER_KEY = "PLACE DEVELOPER KEY HERE" YOUTUBE_API_SERVICE_NAME = "youtube" YOUTUBE_API_VERSION = "v3" def youtube_search(options): youtube = build(YOUTUBE_API_SERVICE_NAME, YOUTUBE_API_VERSION, developerKey=DEVELOPER_KEY) # Call the search.list method to retrieve results matching the specified # query term. search_response = youtube.search().list( q=options.q, part="id,snippet", maxResults= options.max_results ).execute() videos = [] channels = [] playlists = [] # Add each result to the appropriate list, and then display the lists of # matching videos, channels, and playlists. for search_result in search_response.get("items", []): if search_result["id"]["kind"] == "youtube#video": videos.append("%s (%s)" % (search_result["snippet"]["title"], search_result["id"]["videoId"])) elif search_result["id"]["kind"] == "youtube#channel": channels.append("%s (%s)" % (search_result["snippet"]["title"], search_result["id"]["channelId"])) elif search_result["id"]["kind"] == "youtube#playlist": playlists.append("%s (%s)" % (search_result["snippet"]["title"], search_result["id"]["playlistId"])) print "Videos:\n", "\n".join(videos), "\n" print "Channels:\n", "\n".join(channels), "\n" print "Playlists:\n", "\n".join(playlists), "\n" if __name__ == "__main__": argparser.add_argument("--q", help="Search term", default="Google") argparser.add_argument("--max-results", help="Max results", default=25) args = argparser.parse_args() try: youtube_search(args) except HttpError, e: print "An HTTP error %d occurred:\n%s" % (e.resp.status, e.content)Make sure you have replaced the DEVELOPER KEY with your key.
Thanks to developers.google.com
Now you can run this from your terminal to get the videos, channels and playlist from the search query.
Make sure that you should pass the argument from the terminal as python filename.py --pythonTutorialSearch so that it can search on your query else there is a by default case which is embedded.
Thanks :)